ex)
//Objects
//one of the JavaScript's data types.
//a collection of related data and/or functionality.
//Nearly all objects in JavaScript are instances of Object
//object = {key : value};
//1.Literals and properties
const name = 'han';
const age = 4;
print(name, age);
function print(name, age){
console.log(name);
console.log(age);
}
const jin = {name: 'jin', age:27};
function print(person){
console.log(person.name,person.age);
}
print(jin);
//가능한 이렇게 코딩하지 말것
jin.hasJob = true;
console.log(jin.hasJob);
delete jin.hasJob;
console.log(jin.hasJob);
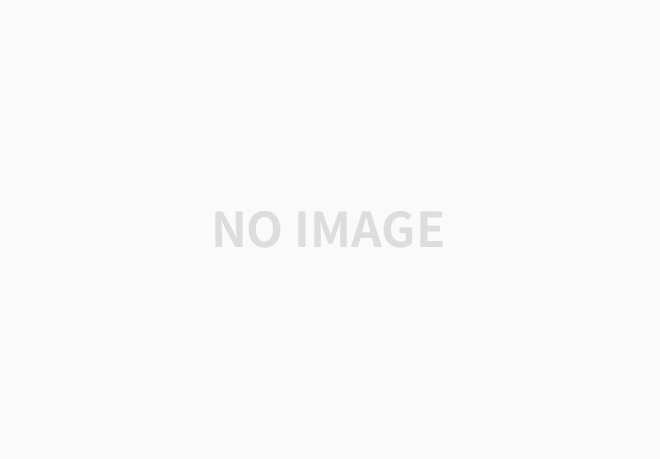
ex)
//2. Computed properties
//key should be always string
const jin = {name: 'jin', age:27};
console.log(jin.name);
console.log(jin['name']);
jin['hasJob'] = true;
console.log(jin.hasJob);
function printValue(obj, key){
console.log(obj.key);
console.log(obj[key]);
}
printValue(jin,'name');
printValue(jin,'age');
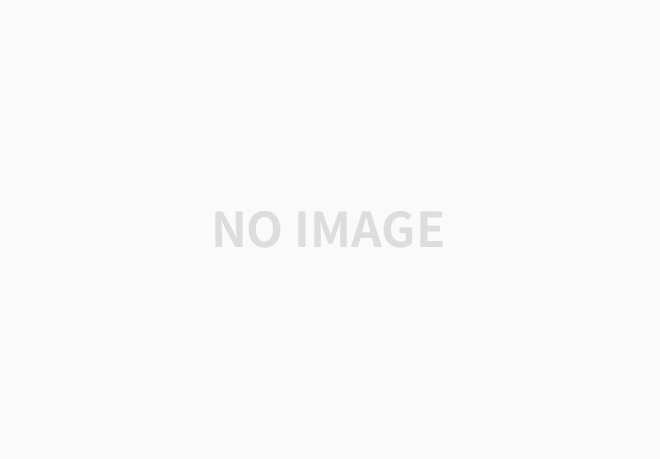
ex)
//3.Property value shorthand
const person1 = {name: 'bob', age: 2};
const person2 = {name: 'steve', age: 3};
const person3 = {name: 'dave', age: 4};
const person4 = new Person('jin', 30);
console.log(person4);
//4. Constructor Function
function Person(name, age){
//this = {};
this.name = name;
this.age = age;
//return this;
}
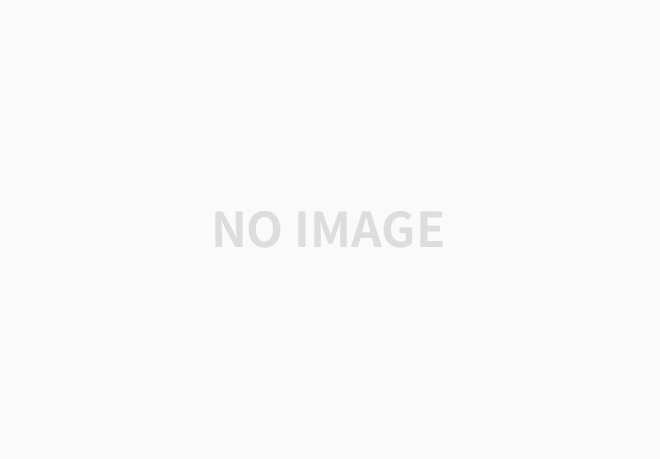
ex)
//5. in operator: property existence check (key in obj)
const jin = {name: 'jin', age:27};
console.log('name' in jin);
console.log('random' in jin);
console.log(jin.random);
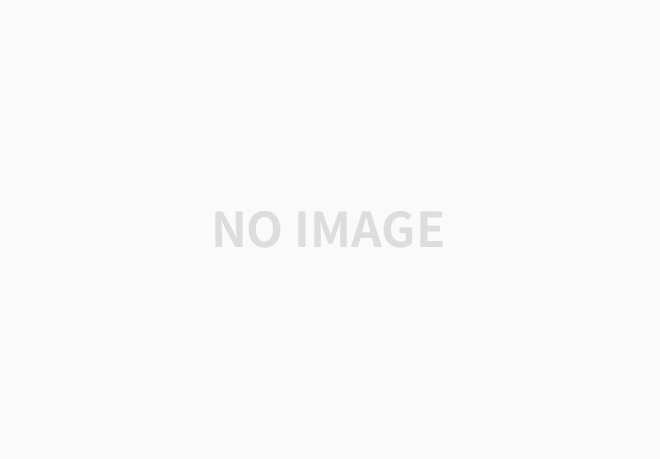
ex)
//6. for..in vs for..of
//for(key in obj)
const jin = {name: 'jin', age:27};
for(key in jin){
console.log(key);
}
//for(value of iterable)
const array = [1,2,4,5];
for(let i = 0; i < array.length; i++){
console.log(array[i]);
}
for(value of array){
console.log(value);
}
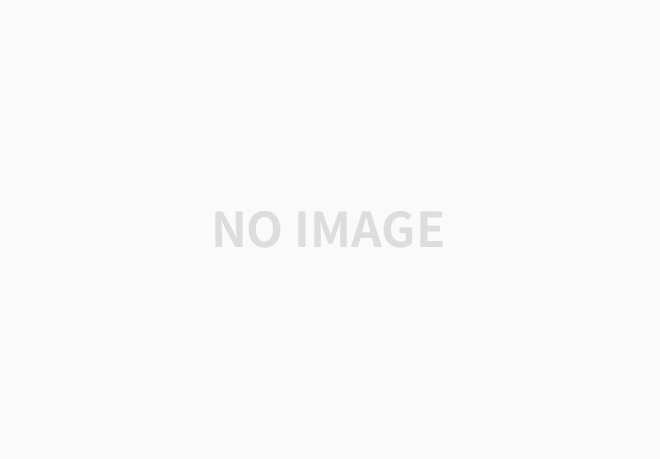
ex)
//7. Fun cloning
//Object.assign(des, [obj1, obj2, obj3...])
const user = {name: 'jin', age: '20'};
const user2 = user;
user2.name = 'han';
console.log(user);
//old way
const user3 = {};
for(key in user){
user3[key] = user[key];
}
console.log(user3);
const user4 = Object.assign({}, user);
console.log(user4);
//another example
const fruit1 = {color: 'red'};
const fruit2 = {color: 'blue', size: 'big'};
const mixed = Object.assign({},fruit1, fruit2); // 같은 key는 뒤로 가면서 계속 덮어쓰기함
console.log(mixed.color);
console.log(mixed.size);
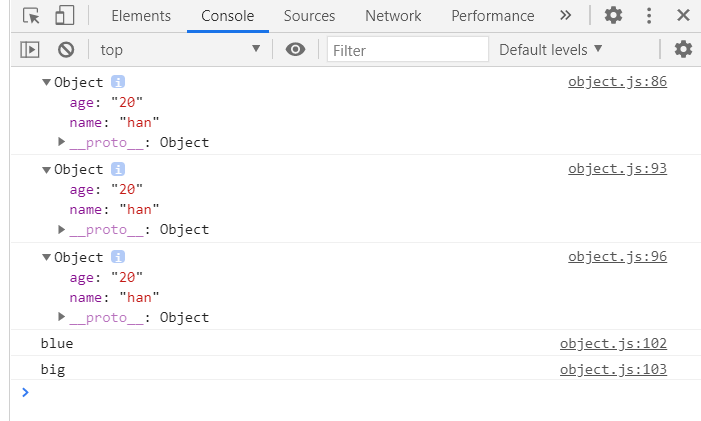
'인터넷강의 > 자바스크립트' 카테고리의 다른 글
유용한 10가지 배열 함수들. Array APIs 총정리 (0) | 2020.09.15 |
---|---|
배열 제대로 알고 쓰자. 자바스크립트 배열 개념과 APIs 총정리 (0) | 2020.09.15 |
클래스와 오브젝트의 차이점(class vs object), 객체지향 언어 클래스 정리 (0) | 2020.09.14 |
Arrow Function은 무엇인가? 함수의 선언과 표현 (0) | 2020.09.14 |
코딩의 기본 operator, if, for loop, 코드리뷰 팁 (0) | 2020.09.11 |