using System;
delegate int CalcDelegate(int x, int y);
delegate void WorkDelegate(char arg1, int arg2, int arg3);
class MessageMap
{
public char opCode;
public CalcDelegate Calc;
public MessageMap(char opCode, CalcDelegate Calc)
{
this.opCode = opCode;
this.Calc = Calc;
}
}
public class Mathematics
{
MessageMap[] aMessageMap;
static int Add(int x, int y) { return x + y; }
static int Subtract(int x, int y) { return x - y; }
static int Multiply(int x, int y) { return x * y; }
static int Divide(int x, int y) { return x / y; }
public Mathematics()
{
aMessageMap = new MessageMap[] {new MessageMap('+', Add), new MessageMap('-', Subtract)
,new MessageMap('*', Multiply), new MessageMap('/', Divide)};
}
public void Calculate(char opCode, int operand1, int operand2)
{
Console.Write(opCode + " : ");
foreach (MessageMap Temp in aMessageMap)
{
if(Temp.opCode == opCode)
{
Console.WriteLine(Temp.Calc(operand1, operand2));
}
}
}
}
class Program
{
static void Main(string[] args)
{
Mathematics math = new Mathematics();
WorkDelegate work = math.Calculate;
work('+', 10, 5);
work('-', 10, 5);
work('*', 10, 5);
work('/', 10, 5);
}
}
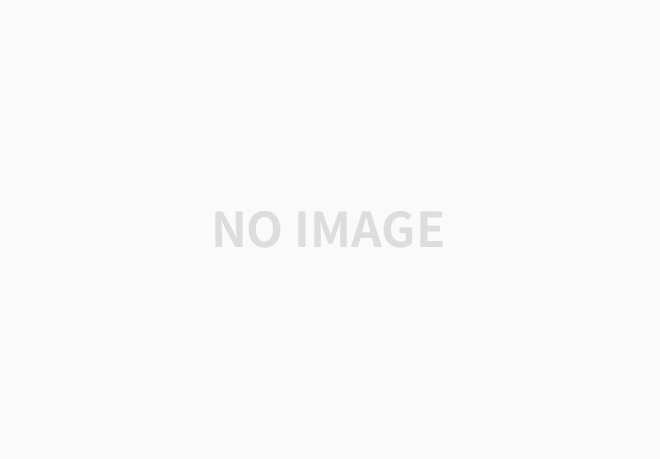
'스마트팩토리 > C#' 카테고리의 다른 글
27. 네트워크 통신 (0) | 2020.07.29 |
---|---|
26. 연산 범위 확인, 가변 매개변수, extern, unsafe (0) | 2020.07.27 |
24. 복습 (0) | 2020.07.24 |
23. Indexer (0) | 2020.07.23 |
22. 복습 (0) | 2020.07.20 |